//The program for CS5532-ASZ
//This is a 24bit ADC and PGIA
//Made by OurWay and 2006/03/21
//#include //根据实际情况定义 //sbit ACC7 = ACC^7; //#define BYTE unsigned char //The ADC results varibles define //The CS5532-ASZ comm define //=== Offset Register === //=== Gain Register === //=== Configuration Register === #define PSS 0x80 //Power Save Select //Channel Select Bits //=== Converter mode === //The data(8bit) form MCU to CS5532 //The Setup CS5532's register //The data(8bit) form CS5532 to MCU //Receive ADC signal data form CS5532 to MCU #if ReadMADC5532Run //Receive CS5532's Register from CS5532 to MCU #if Adjust5532Run //The initialization CS5532 //用的时间注意我定义的宏,这是个查询方式采集AD值的程序。
//#include
//sbit SDI5532 = P2^1;
//sbit SDO5532 = P2^2;
//sbit CLK5532 = P2^3;
//sbit CS5532 = P2^0;
//sbit ACC0 = ACC^0;
//#define WORD unsigned int
#define Adjust5532Run 0
#define ReadSADC5532Run 1
#define ReadMADC5532Run 1
struct{
unsigned char top;
unsigned char high;
unsigned char mid;
unsigned char low;
}
RegDat;
#define RegRead 0x08
#define RegWrite 0x00
#define OffsetRS 0x09
#define GainRS 0x0a
#define ConfigWrite 0x03 //write config
#define ConfigRead 0x0b //read config
#define PDW 0x40 //Power Down Mode
#define RS 0x20 //Reset System
#define RV 0x10 //Reset Valid
#define IS 0x08 //Input Short
#define GB 0x04 //Guard Signal Bit
#define VRS 0x02 //Voltage Reference Select(Ref>2.5V,VRS=0)
#define A1 0x01
#define A0 0x80
#define OLS 0x40
#define OGS 0x10
#define FRS 0x08
//=== Channel Setup Register ===
#define SetupCH1 0x05
#define SetupCH2 0x15
#define CH1 0x00 //CS1=0,CS0=0
#define CH2 0x40 //CS1=0,CS0=1
//Gain Bits
#define Gain1 0x00
#define Gain2 0x08
#define Gain4 0x10
#define Gain8 0x18
#define Gain16 0x20
#define Gain32 0x28
#define Gain64 0x30
#define SingleC 0x80
#define ContinC 0xC0
#define Setup1 0x00
#define Setup2 0x08
#define Setup3 0x10
#define Setup4 0x18
#define Setup5 0x20
#define Setup6 0x28
#define Setup7 0x30
#define Setup8 0x38
void SendByte5532(unsigned char Dat)
{
unsigned char i;
CLK5532 = 0;
for(i=8;i>0;i--)
{
SDI5532=(bit)(Dat & 0x80);
CLK5532=1;
_nop_();_nop_();
_nop_();_nop_();
CLK5532=0;
_nop_();_nop_();
_nop_();_nop_();
Dat = Dat<<1;
}
SDI5532 = 1;
}
void WriteReg5532(BYTE command,BYTE low,BYTE mid,BYTE high,BYTE top)
{
CS5532 = 0;
SendByte5532(command);
SendByte5532(low);
SendByte5532(mid);
SendByte5532(high);
SendByte5532(top);
CS5532 = 1;
}
unsigned char ReceiveByte5532(void)
{
unsigned char i;
ACC=0;
for(i=8;i>0;i--)
{
ACC=ACC<<1;
ACC0=SDO5532;
CLK5532=1;
_nop_();_nop_();
_nop_();_nop_();
CLK5532=0;
_nop_();_nop_();
_nop_();_nop_();
}
return(ACC);
}
#if ReadSADC5532Run
void ReadSADC5532(unsigned char command)
{
CS5532 = 0;
SendByte5532(command);
do{_nop_();CLK5532=0;SDI5532=0;}while(SDO5532!=0);
SendByte5532(0x00); //8bit SCLK and SDI=0;
RegDat.top = ReceiveByte5532();
RegDat.high = ReceiveByte5532();
RegDat.mid = ReceiveByte5532();
RegDat.low = ReceiveByte5532();
CS5532 = 1;
}
#endif
void ReadMADC5532(unsigned char command)
{
CS5532 = 0;
do{_nop_();}while(SDO5532!=0);
//SDO5532 = 1;
SendByte5532(command); //8bit SCLK and SDI=command;
RegDat.top = ReceiveByte5532();
RegDat.high = ReceiveByte5532();
RegDat.mid = ReceiveByte5532();
RegDat.low = ReceiveByte5532();
CS5532 = 1;
}
#endif
void ReadReg5532(unsigned char command)
{
CS5532 = 0;
SendByte5532(command);
RegDat.top = ReceiveByte5532();
RegDat.high = ReceiveByte5532();
RegDat.mid = ReceiveByte5532();
RegDat.low = ReceiveByte5532();
CS5532 = 1;
}
void Adjust5532(unsigned char command)
{
CS5532 = 0;
SendByte5532(command);
do{_nop_();}while(SDO5532!=0);
SendByte5532(0x0a);
RegDat.top = ReceiveByte5532();
RegDat.high = ReceiveByte5532();
RegDat.mid = ReceiveByte5532();
RegDat.low = ReceiveByte5532();
CS5532 = 1;
}
#endif
void Init5532(void)
{
WriteReg5532(0xff,0xff,0xff,0xff,0xff);
WriteReg5532(0xff,0xff,0xff,0xff,0xff);
WriteReg5532(0xff,0xff,0xff,0xff,0xff);
WriteReg5532(0xff,0xff,0xff,0xff,0xfe);
}
//The CS5532-ASZ subpram end
上一篇:data,bdata,idata,pdata,xdata,code存储类型与存储区
下一篇:手把手教你学51单片机-点亮LED
推荐阅读最新更新时间:2024-11-07 10:36
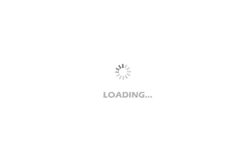
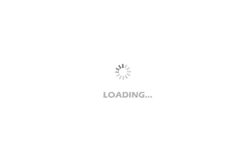
设计资源 培训 开发板 精华推荐
- LTC3526LEDC-2 2 节电池至 5V 升压转换器的典型应用电路
- AD688 高精度 10V 基准的典型应用电路,用于通过降噪进行增益和平衡调整
- AC103011,PIC10F32x 开发板,适用于对 PIC MCU 设计和开发应用程序感兴趣的个人
- 使用 Analog Devices 的 LTM8003-3.3IY 的参考设计
- LT1117CM-3.3 1.2V 至 10V 可调稳压器的典型应用
- NCS2001SN1T1G 0.9V 单电源运放可变占空比脉冲发生器的典型应用电路
- 4S 21700保护板
- LT1764ET-1.8 并联 LDO 稳压器以实现更高输出电流的典型应用
- SC630A 1MHz 固定 3.3V 输出电荷泵稳压器的典型应用
- DC1956A,使用具有 APD 电流监视器的 LT3905 升压 DC/DC 转换器的演示板